Integration Testing and Unit Testing in the Age of AI
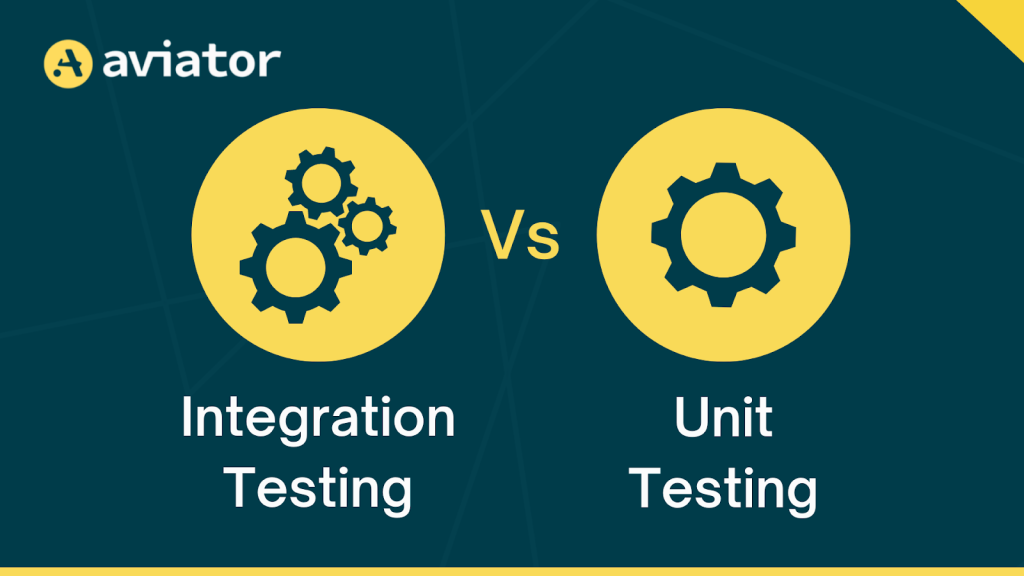
With the popularity of “shift-left” movement and the proliferation of AI-based tools, testing landscape is evolving quickly. But with so many types of testing, how do you know when to use unit tests versus integration tests?
AI tools can now speed up the process by generating both unit and integration tests, but knowing when and how to apply them is still essential. In this blog, we’ll dive into the differences between unit and integration testing, look at how AI has influenced testing, provide hands-on examples, share best practices, and help you decide when to apply each method based on your application’s needs.
By the end, you’ll have a clear understanding of how these two approaches can work together to strengthen your development process and improve your application’s reliability. Whether it’s ensuring testing at the initial phase or managing to pull requests for your source code, understanding when and how to apply these tests is key to a smooth development process.
What is Unit Testing?
Unit testing is the process of testing individual units, functions, or methods in isolation. It focuses on validating that a single component of your code behaves as expected without relying on external dependencies or other pieces of code. This basic testing ensures that every single unit performs correctly before moving on to more complex forms of testing like integration or acceptance testing.
Unit testing offers several advantages, such as:
- Early Detection of Bugs: Unit testing helps identify bugs early in the software development process, making them easier and cheaper to fix, especially when dealing with tests from integration tests later on.
- Simplifies Debugging: When a test fails, it pinpoints the exact location of the issue within the internal design, providing detailed visibility and making debugging faster. This kind of white-box testing approach allows developers to dig deeper into the source code and fix problems faster.
- Improved Code Quality: Focusing on testing small, individual modules leads to cleaner, more maintainable code.
- Acts as Documentation: Unit tests provide clear examples of how each part of the system should behave. They serve as functional tests that help developers understand the interface specification of each module.
- Cost-Effective Testing: Automated unit tests reduce time and resources spent on manual testing, resulting in faster test execution and making the process more efficient and less reliant on external dependencies.
When to Use Unit Testing?
Unit testing is especially useful in projects where frequent updates are made, as basic tests help catch bugs early and ensure new changes don’t break existing functionality. Unit tests work best for pieces of code with clear inputs and outputs, making it easy to cover multiple scenarios and edge cases quickly.
In projects using Test-Driven Development (TDD), unit testing plays an important role. TDD is when developers write tests before the actual code, guiding them to build code that meets specific needs right from the start. This approach encourages reliable, testable code at the unit level, catching issues early and improving overall code quality.
For example, in an e-commerce site, a unit test can verify that a function calculating the total price of items in a shopping cart, including discounts and taxes, works correctly. By isolating and testing this function, you can ensure it handles different pricing scenarios without needing to run the entire checkout process. This approach allows developers to confidently make changes to the function, knowing that any errors in the logic will be caught early.
When Not to Use Unit Testing?
While unit testing is valuable for testing isolated functions, there are times when it may not be the best approach:
- Rapid Prototyping: If you’re working on code that’s likely to be discarded or rewritten, like in early prototyping, unit tests may not be worth the effort.
- Simple, Obvious Code: For straightforward code with minimal logic, such as getters, setters, or basic data structures, unit tests may not add much value.
- UI Rendering Details: Visual elements and layout changes are better tested through UI or end-to-end tests rather than unit tests, as they involve user interaction.
- Infrastructure / IaC: Infrastructure code, such as cloud configurations or IaC (Infrastructure as Code), is better suited for integration tests to ensure everything works in the actual environment.
- Database Migrations: Testing database migration scripts or schema changes often requires integration testing to confirm they work well with the actual database.
In these cases, integration testing or end-to-end testing will give you a more comprehensive understanding of how the system behaves as a whole.
Hands-on Example: Using GitHub Copilot AI to Write Unit Tests in JavaScript
Let’s see how GitHub Copilot can help us quickly generate unit tests for a simple function. We’ll create a password validator function that checks the strength of a password and, with the assistance of Copilot, generates unit tests.
Step 1: Define the Password Validator Function
Let’s create a basic function that validates passwords based on specific criteria, such as length, uppercase, lowercase, and numbers.
// passwordValidator.js
function validatePassword(password) {
const hasUpperCase = /[A-Z]/.test(password);
const hasLowerCase = /[a-z]/.test(password);
const hasNumber = /\d/.test(password);
const minLength = password.length >= 8;
return hasUpperCase && hasLowerCase && hasNumber && minLength;
}
module.exports = validatePassword;
This function checks if the password meets the following criteria:
- Contains at least one uppercase letter
- Contains at least one lowercase letter
- Contains at least one number
- Has a minimum length of 8 characters
Step 2: Generate Unit Tests with GitHub Copilot
Now, we’ll use Copilot to generate unit tests for the validatePassword function. Open a new file, passwordValidator.test.js, and type a comment to guide Copilot:
// passwordValidator.test.js
// Write unit tests for the validatePassword function
After typing the comment, Copilot will suggest test cases. Here’s an example of a prompt and tests generated by Copilot.
Example Prompt
Type the following prompt to guide Copilot in generating useful tests:
“Write a test case to validate that the password contains at least one uppercase letter, one lowercase letter, one number, and is at least 8 characters long.”
In this case, Copilot generated the following test cases based on this prompt:
const validatePassword = require('./passwordValidator');
test('validates password with all criteria met', () => {
expect(validatePassword('StrongPass1')).toBe(true);
});
test('fails password without uppercase letter', () => {
expect(validatePassword('weakpass1')).toBe(false);
});
test('fails password without lowercase letter', () => {
expect(validatePassword('WEAKPASS1')).toBe(false);
});
test('fails password without a number', () => {
expect(validatePassword('WeakPass')).toBe(false);
});
test('fails password with less than 8 characters', () => {
expect(validatePassword('WkP1')).toBe(false);
});
Step 3: Run the Tests
Once your tests are ready, run them using Jest by typing this in your terminal:
npx jest
If everything is set up correctly, Jest will run the test and show that it passes:
PASS ./passwordValidator.test.js
✓ validates password with all criteria met (5 ms)
✓ fails password without uppercase letter (3 ms)
✓ fails password without lowercase letter (2 ms)
✓ fails password without a number (3 ms)
✓ fails password with less than 8 characters (3 ms)
By integrating AI tools like GitHub Copilot, developers can accelerate the process of writing unit tests, focusing more on refining logic rather than manually creating each test case.
What is Integration Testing?
While unit testing focuses on testing individual units of code in isolation, integration testing comes into play when you need to verify how integrated units of the system work together. Instead of testing each component on its own, integration tests check how they interact to ensure the overall system functions as expected. These tests are a key part of various types of testing strategies used in complex systems.
Integration testing offers several advantages, such as:
- Catches Interaction Issues: It helps identify bugs in component interactions, ensuring smooth operation early in the testing process. This may include interaction with external dependencies like APIs or services, ensuring that the integration works well.
- Ensures Correct Data Flow: Verifies that data moves properly between application parts, preventing unexpected behavior. This is crucial when dealing with Functional testing or E2E tests, where a failure in data flow could lead to larger system issues.
- Validates Component Communication: Confirms that different parts of the system communicate and function smoothly together, especially in real-world business scenarios. This includes checking the public interface and ensuring correct handling of integration testing checks during critical workflows.
- Identifies External Integration Errors: Detects issues when integrating with external resources or APIs within the system.
- Reduces Complex Integration Risks: Helps minimize risks by testing component interactions in complex systems. This ensures that the system is adequately tested for data consistency and performance.
When to Use Integration Testing?
Integration testing is useful when different components interact closely and rely on each other to complete a workflow. It helps catch integration-related issues such as data flow errors or miscommunication between services, making it a key part of the testing pyramid.
For example, in an e-commerce site, integration testing can check how the checkout process works by testing how the shopping cart, payment gateway, and inventory system interact. This ensures that when a user places an order:
- The payment is processed,
- The inventory is updated,
- An order confirmation is generated.
By testing these interactions, you reduce the chance of issue escape and ensure the system produces the actual output as expected.
When Not to Use Integration Testing
While integration testing is essential for ensuring different components work together, it may not be the right choice in certain cases:
- Unstable External Dependencies: If external systems are unreliable, integration tests can become flaky and unreliable. Unit tests can help by isolating and testing core functionality without depending on these unstable systems.
- Rapid Prototyping: For quick prototypes or exploratory code that may be discarded, integration testing can be excessive. Focus on simple checks and flexibility to keep development efficient.
- UI Appearance Validation: Integration tests aren’t suitable for visual details like layout or design consistency. Visual regression tests are better for catching appearance issues across updates.
- Isolated Component Testing: Integration tests are unnecessary when you only need to check the behavior of a single, self-contained function or module. Unit testing is more efficient for such scenarios.
In these cases, unit testing is more efficient for quickly testing individual functions or simple logic without involving multiple components.
Hands-on Example: Using GitHub Copilot AI for Integration Testing in Node.js
In this example, we’ll create a simple URL shortener REST API and use GitHub Copilot to help us write an integration test to verify that the controller and service work together correctly. The API will take a full URL as input and return a shortened version.
Step 1: Define the URL Shortener Service
We’ll start by defining a service that handles the URL shortening logic.
// urlService.js
function shortenURL(url) {
return `short.ly/${Math.random().toString(36).substring(7)}`; // Generates a short, random URL
}
module.exports = shortenURL;
This service function takes a URL as input and returns a randomly generated short URL.
Step 2: Create the Controller
Next, we’ll create a controller that uses the shortenURL service to handle API requests.
// urlController.js
const shortenURL = require('./urlService');
function urlController(req, res) {
const { url } = req.query;
if (!url) {
return res.status(400).json({ error: "URL is required" });
}
const shortUrl = shortenURL(url);
res.json({ originalUrl: url, shortUrl });
}
module.exports = urlController;
The controller reads a URL from the query parameters, calls shortenURL, and returns both the original and shortened URLs as a JSON response.
Step 3: Define the Route
We’ll set up an Express route that uses the controller to handle requests.
// app.js
const express = require('express');
const urlController = require('./urlController');
const app = express();
app.get('/shorten', urlController);
module.exports = app;
This code sets up a /shorten endpoint where users can provide a URL to get a shortened version.
Step 4: Write the Integration Test with GitHub Copilot
Now, we’ll use GitHub Copilot to generate an integration test that verifies the /shorten endpoint by testing the interaction between the controller and service.
Open a new file, url.test.js, and type the following comment to guide Copilot:
// url.test.js
// Write an integration test for the /shorten API endpoint
After typing the comment, Copilot will suggest a test code for the /shorten endpoint. Here’s an example of a prompt and test generated by Copilot.
Example Prompt
Here’s an example prompt to guide Copilot in generating a useful test:
“Write a test to verify that the /shorten endpoint returns a short URL for a valid input URL.”
In our case, using this prompt Copilot generated the following test case:
const request = require('supertest');
const app = require('./app');
describe('GET /shorten', () => {
it('should return a shortened URL for a valid input', async () => {
const response = await request(app).get('/shorten?url=http://example.com');
expect(response.statusCode).toBe(200);
expect(response.body).toHaveProperty('originalUrl', 'http://example.com');
expect(response.body.shortUrl).toMatch(/^short\.ly\//); // Checks if the short URL is in the correct format
});
it('should return a 400 error if no URL is provided', async () => {
const response = await request(app).get('/shorten');
expect(response.statusCode).toBe(400);
expect(response.body).toHaveProperty('error', 'URL is required');
});
});
Step 5: Run the Test
To run the test, use this command:
npx jest
If everything is set up correctly, Jest will run the test and show that it passes:
PASS ./url.test.js ✓ should return a shortened URL for a valid input (20 ms) ✓ should return a 400 error if no URL is provided (10 ms)
Breaking It Down: Key Differences Between Unit and Integration Testing
Now that we’ve gone over unit and integration testing, let’s look at how they differ. This comparison will help you understand what each testing method does and when it’s best to use them in your development process.
Metric | Unit Testing | Integration Testing |
Focus Area | Focuses on testing individual components in isolation | Tests how multiple components work together as a system |
Goal | Ensures individual functions or methods work correctly using functional tests | Validates the interaction between integrated components and database for tests |
Speed and Complexity | Quick to execute and relatively simple | Slower execution and more complex due to multiple components |
Tools and Technologies | Common tools: Jest, Mocha, JUnit | Common tools: Wiremock, JUnit, Supertest |
AI Effectiveness | Highly effective: AI can quickly generate tests for isolated functions with minimal adjustments needed. | Limited effectiveness: AI-generated integration tests often need more review to ensure accuracy in interactions. |
Real-world Scenario | Testing a function that validates an email format | Testing how a registration form integrates with a backend |
Execution Time | Fast, it runs on isolated components. | Comparatively slower, it tests multiple interacting components |
Resource Requirements | Low resource usage and minimal setup | It may require more resources and has a more complex setup |
Visibility | Offers in-depth code exposure | Provides a detailed view of component interactions |
Best Practices for Testing and Integrating with CI/CD
To get the most out of unit and integration testing, it’s essential to follow best practices that improve both the speed and effectiveness of your tests:
- Write Tests Early: Start unit testing as you develop new features, following test-driven development principles. This catches bugs early and ensures everything works as expected from the start. Early testing also allows the testing team to find and fix issues before they become more complex.
- Test Key Interactions: Use integration tests to check important workflows, like login or payments. Focus on important interactions where components need to work together. This helps ensure that your system handles complex issues related to the integration of modules.
- Keep Tests Simple: Write small, clear tests that are easy to maintain. Simple tests help quickly spot issues and keep your testing process smooth. Avoid overcomplicating things by sticking to clear forms of testing that focus on the essentials.
- Stub External Services: For integration testing, test components within a service and stub external calls. This keeps tests focused, faster, and free from dependency on external systems, allowing for better functionality testing.
- Automate Testing: Add both unit and integration tests to your CI/CD pipeline. Automating these tests ensures that bugs are caught before deployment, saving time and making sure the system is stable. Automation also helps run tests at regular intervals, reducing the chance of issue escape during production.
Example
Here’s an example of how unit and integration tests can work together in a CI/CD pipeline for an e-commerce platform:
First, you write unit tests for individual components, such as the price calculation function in the shopping cart. These tests ensure:
- The total price, including discounts and taxes, is calculated correctly.
- The function handles different scenarios, like varying product quantities and discount types.
- Any errors are caught early, ensuring the function works as expected in code in isolation.
After the unit tests pass, you move to integration tests that focus on the interaction between a few key components. These tests might check:
- If the order is correctly processed after the checkout is completed.
- If the payment gateway successfully processes the payment and updates the order status.
- Whether the inventory system correctly adjusts stock levels after an order is placed.
By combining both unit testing and integration tests, you ensure:
- Each individual function, like price calculation, works correctly in isolation.
- The interactions between components like checkout, payment, and inventory are functioning as expected, handling real dependencies without testing the full system.
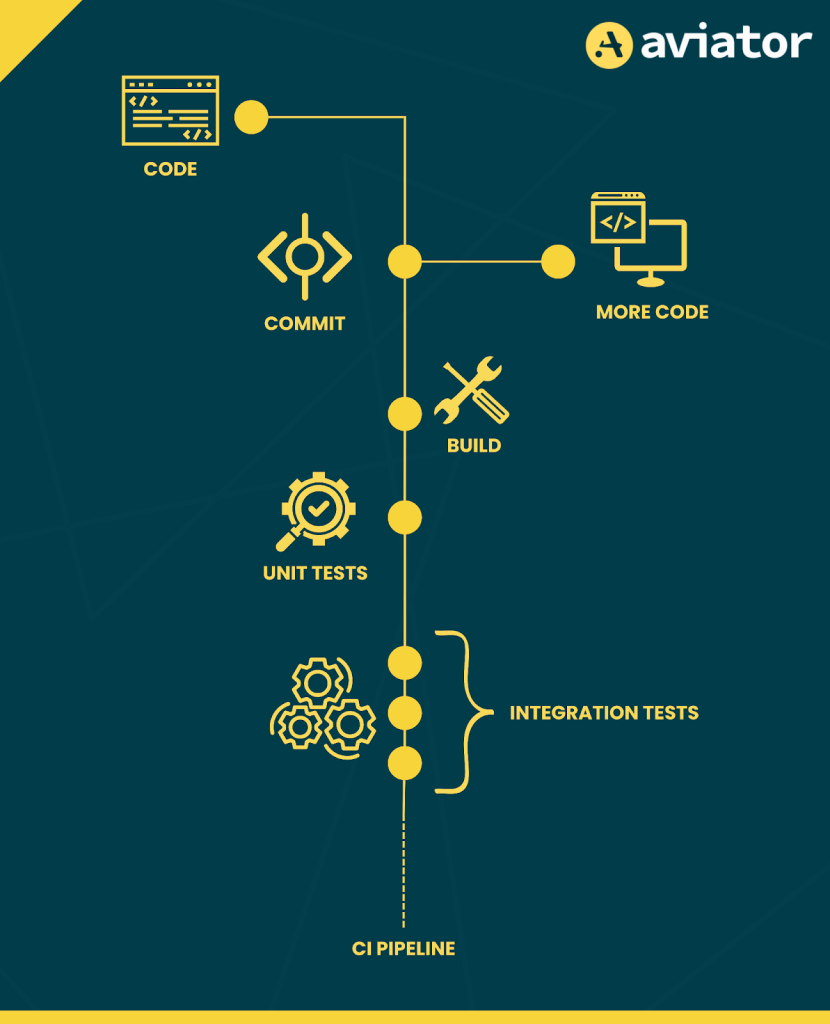
This approach ensures that isolated components and their core interactions work smoothly, allowing you to catch bugs early and ensure the system performs well in production.
Why Focusing on Code Coverage Isn’t Enough
Code coverage is often used as a metric to see how much of the code is being tested, but relying too much on code coverage numbers can be misleading. Instead of aiming for high coverage, it’s more important to focus on the quality of the tests themselves. Here’s why:
- Coverage Doesn’t Equal Quality: High coverage can miss critical bugs if tests aren’t thorough.
- False Confidence: High coverage can make you feel secure, but weak tests may still pass.
- Real-World Bugs Matter: Tests should focus on real use cases rather than covering every line of code.
- Time-Efficient Testing: Prioritize testing important code paths over chasing full coverage.
- Quality Over Quantity: Fewer meaningful tests are better than lots of shallow ones.
In short, while code coverage helps, the real focus should be on writing tests that catch real issues and cover critical functionality.
Wrapping It Up: Key Takeaways
We’ve covered how unit tests help catch early bugs by testing individual components, while integration tests ensure different parts of the system work well together. We’ve also covered how AI tools like GitHub Copilot make writing tests easier, especially for unit testing, and adding tests to your CI/CD pipeline helps catch issues before they reach production. Following best practices and applying these tests effectively ensures smooth development and long-term stability.
By combining both unit and integration testing, you get a complete testing approach. As your project grows, continue refining your tests to cover new features, ensuring long-term reliability and adequately testing the system.
Frequently Asked Questions
1. What is the difference between integration testing and unit testing?
Unit testing checks individual components, like functions or methods, to ensure they work on their own. These tests are often part of white-box tests where you have visibility into the internal design. Integration testing, on the other hand, focuses on how these units of code work together. It tests interactions between parts of a software application to catch issues that happen when they’re combined, making it a key part of your testing strategies.
2. What is an example of integration testing?
A good example of integration testing would be checking how an e-commerce app’s checkout process works. The test would ensure that when a user adds items to the cart, the payment service processes the transaction, and the inventory system updates the stock levels accordingly.
3. Is API testing integration testing?
API testing can be a type of integration testing. It tests how your application interacts with an external API, ensuring that the data exchange between your app and the API works correctly and that your components handle the API responses as expected. This can be considered a form of black box testing technique because you’re testing the external behavior without focusing on the internal code.
4. Do integration tests replace unit tests?
No, integration tests don’t replace unit tests. Unit tests verify specific functions or methods in your source code, while integration tests focus on how components work together. Both are important, serve different purposes, and help catch bugs early at different stages of the testing process, including acceptance level and basic tests. Unit tests may run faster, helping with faster test execution, while integration tests provide a broader scope by focusing on integration testing checks.