Technical Debt and the Role of Refactoring
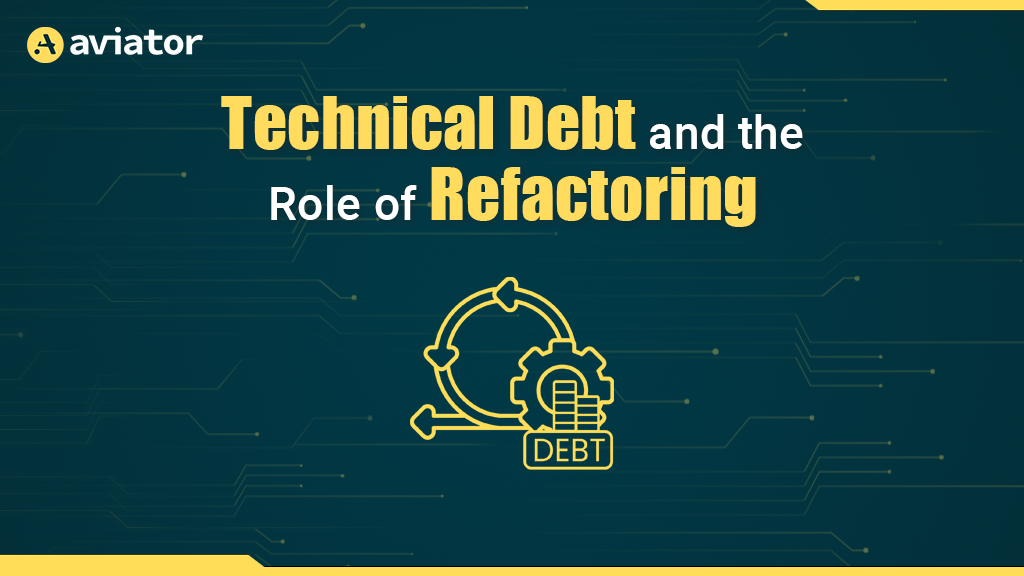
Businesses working in software development tend to prioritize speed over optimization during rapid development phases. Releasing code quickly to hit deadlines leads teams to create inefficiencies in their apps, resulting in technical debt. Similar to financial debt, Technical debt leads to increased development costs and reduces future development speed. Technical debt without management converts into significant obstacles that affect performance, decreasing system scalability and software quality.
That’s where Refactoring can help teams. Refactoring helps in rebuilding and optimizing the fundamental structure of an existing programming code without modifying its operational capabilities. However, refactoring is neither a one-time process nor a one-size-fits-all solution. Cutting short on quality over early delivery often leads to backlogs in the corner. For example, startups in the seed stage have an idea, and they rush to implement those ideas and features, often cutting corners to launch quickly. They commit to refactoring things later on, but that never happens as they get involved in handling customer issues or adding new features.
Over time, the code becomes messy, and frustration grows as founders wonder why the development has slowed down so much. Even if the startup gets acquired, most of its real value was created in the first few years. The later years are spent struggling with technical debt rather than driving meaningful growth. So, the question is about understanding technical debt and its impact on businesses and startups and how continuous refactoring is asolution for controlling it.
Technical Debt in Software Development: What You Need to Know
What is Technical Debt?
Technical debt describes the expected future costs of maintenance that occur when developers choose fast substandard solutions over sustainable practices during current software development.
Software developer Ward Cunningham introduced technical debt as he connected it to loan borrowing principles—just like taking out business loans enables initial momentum with future interest payments for repayment.
Suppose a team rushes to deliver a product by skipping comprehensive testing or writing poorly structured code; they incur technical debt. While this may provide short-term gains, it leads to long-term consequences such as higher bug-fixing efforts, difficulty in scaling, and slower feature development.
Let’s see a code example: Hardcoded Configuration Instead of Using Environment Variables.
def connect_to_database(): # Hardcoded credentials – bad practice db_host = “localhost” db_user = “admin” db_password = “password123” # Storing sensitive info in code print(f”Connecting to database at {db_host} with user {db_user}”) connect_to_database() |
This is a quick solution that works fine locally but can cause problems in production because changing credentials requires modifying the source code, increasing the risk of exposing sensitive data.
What Are the Different Types of Technical Debts?
Technical debt can be Intentional and Unintentional, as first introduced by Steve McConnell in 2007. Martin Fowler further expanded on this concept, highlighting that not all technical debt is bad. Some can be strategic if managed properly, while unmanaged debt can cripple future development.
Let’s suppose your team approaches a significant product release deadline with heavy time constraints.
During testing, you realise that a configuration value–say, an API’s endpoint or a feature flag should adapt dynamically, but it isn’t working as expected. Since a proper configuration management system is not in place, you decide to hardcode the value straight into the code to meet your deadline.
Now, this is a type of intentional technical debt, where you knowingly make a strategic choice or shortcut for the sake of speed and plan to fix it later. However, if you leave this unaddressed, this hardcoded value may create problems across environments, making future updates more complex and increasing maintenance efforts.
On the other hand, unintentional technical debt is not planned and is a result of developers creating a temporary solution due to time constraints without realising its long-term impact. For example, instead of refactoring an inefficient database query, they add an index in one place, unknowingly causing performance bottlenecks elsewhere.
The Technical Debt Quadrant
Looking further, Martin Fowler expanded on McConnell’s ideas. He categorized technical debt into four major types based on two dimensions, each quadrant stating a type:
- Intent ( Deliberate vs. Inadvertent)
- Awareness ( Prudent vs Reckless)
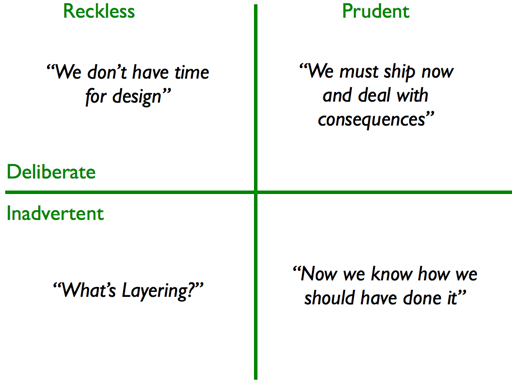
Prudent vs. Reckless Debt: The Real Differentiator
The debt metaphor reminds people of their choices regarding design flaws. Technical debt taken with prudence for release delivery sometimes becomes unnecessary to repay because the maintenance effort or “interest payments” remain affordable, such as in areas of the codebase where modifications rarely occur.
Thus, the key distinction is not simply debt vs. no debt but rather whether the debt is prudent or reckless:
- Prudent debt is strategic. Teams understand precisely what they are getting into by accepting this debt because they have established that future expenses will be easy to handle. Development teams assess the future expenses of debt repayment with the potential benefits of accelerated delivery and release.
- Reckless debts are unplanned and risky. It stems from bad decisions and ignorance toward industry best practices, thus producing major costs that extend over time.
How Should Early-Stage Startups Handle Technical Debt?
For early-stage startups, understanding the difference between prudent and reckless debt is important to managing and planning technical debt. In the initial phases, the main focus is to make a market-fit product and prioritize speed over perfection, so debt is planned intentionally or strategically, and prudence is used for it. Here, teams will acknowledge the trade-offs and plan future fixes.
The key idea is to speed up delivery but not at the cost of quality– we can compromise on optimization later on, but bad quality code and taking shortcuts can result in reckless debts.
The early stages are all about experimenting with products and features, so it’s not a big deal if some debts occur. However, as startups scale, they must shift focus to addressing accumulated debt, ensuring that short-term trade-offs do not compromise long-term growth.
The Difference Between Deliberate and Inadvertent Debt
Beyond identifying reckless debt from prudent debt, let’s look into another important distinction of the technical debt quadrant:
- Deliberate debt occurs when a team decides to take debt while it simultaneously considers both shorter-term release benefits against long-term expenses.
- Inadvertent debt happens when a team lacks both experience and knowledge about accumulating debt.
Reckless debt does not necessarily happen by mistake. The team chooses to dismiss proper design principles by using a fast-but-imperfect strategy instead of clean code because they believe the time needed for proper code creation is unaffordable. Such approaches create technical burdens down the line.
Is Technical Debt Quadrant Sufficient?
In 2014, a group of researchers found that the classification methods for technical debt were insufficient. They developed a classification system built from the core features of technical debt rather than adopting McConnell and Fowler’s strategic methodology.
A research paper by the Software Engineering Institute, “Towards an Ontology of Terms on Technical Debt” detailed 13 technical debt types with different monitoring indicators.
- Architecture Debt
- Build Debt
- Code Debt
- Defect Debt
- Design Debt
- Documentation Debt
- Infrastructure Debt
- People Debt
- Process Debt
- Requirement Debt
- Service Debt
- Test Automation Debt
- Test Debt
Refactoring and Its Role in Debt Reduction
What is Refactoring?
Refactoring is a method that helps professional programmers reorganize code structures for better readability, making them less complex and maintainable while keeping external behaviors unaltered.
Let’s look at an example. We’ll consider the same debt as discussed above: Hardcoded Configuration Instead of Using Environment Variables.
Here’s the refactored version:
import os def connect_to_database(): # Using environment variables – best practice db_host = os.getenv(“DB_HOST”, “localhost”) db_user = os.getenv(“DB_USER”, “admin”) db_password = os.getenv(“DB_PASSWORD”, “password123”) # Default values for safety print(f”Connecting to database at {db_host} with user {db_user}”) connect_to_database() |
Why Refactoring is a Continuous Process, Not a One-Time Fix
In software development, perfection is a moving target. Growth comes at a cost—Enterprises dedicate 41% of their IT funds to handling technical debt, whereas small companies spend 27%. So, to keep up with quality work, regular refactoring is necessary.
A one-time refactoring might seem like a quick fix, but it often disrupts development flow, delaying feature rollouts and increasing stress on teams. Continuous refactoring helps maintain good code quality by making routine and small changes to it. This approach prevents major overhauls, reduces long-term costs, and ensures the code stays flexible as business needs evolve.
Framework for Managing Technical Debt
Let’s now focus on what framework is best for managing technical debt. Here, we’re going to look into the 3 Pillars of Refactoring and how you can use them to manage technical debts:
1. Practices
This pillar focuses on maintainable coding standards that include architectural choices, coding patterns and directory structures for the codebase. If you don’t have practices in place, you don’t really know where you’re going, you’re just refactoring in vain.
Key Aspects:
- Refactoring Patterns: Use techniques like Extract Method, Move Function and Split Large Classes to improve both readability and code maintainability.
- Architecture of Code: Prefer modularization, proper dependency management, and separation of concerns (e.g., implementing microservices to reduce monolithic complexity).
- Folder Structure: Designing consistent file structures (e.g., feature-based structure in React apps, MVC structure in backend frameworks) to boost coding supportability.
2. Inventory
Inventory helps you identify gaps and to know how far you are from your ideal scenario. This pillar will analyse what is missing and prioritize that in the very instance.
Key Aspects:
- Research and Analysis: Tools such as SonarQube and CodeClimate can help with static code analysis and detect areas requiring debt reduction.
- Identifying Gaps: Compare the existing codebase with best practices and determine where refactoring is necessary.
- Define the Target State: Set up benchmarks (eg: maximum acceptable cyclomatic complexity values, test coverage goals.)
3. Process
The execution phase involves implementing refactoring while ensuring proper ownership, tracking, and balancing feature development with technical improvements.
Key Aspects:
- Execution Plan: Refactoring requires a deliberate execution plan that uses incremental changes instead of attempting to complete every change in one large development. You can use refactoring techniques such as:
- Extract Class – Splits a large class into smaller, focused ones.
- Move Method/Field – Relocates logic to the most relevant class.
- Decompose Conditional – Breaks complex conditions into readable methods.
- Use Composition Over Inheritance – Replaces rigid hierarchies with flexible composition.
- Tracking Progress: Use tools like Jira or Linear to monitor the separate progress of refactoring initiatives.
- Knowing When to Stop: Define completion criteria (e.g., achieving a set performance improvement or reaching a maintainability score threshold).
Rules to Make Refactoring Work
A framework will only be effective if you put in the right planning. Let’s see the rules on how you can integrate it into your regular development flows:
1. Make It Visible – Define What Needs Refactoring
Check what gaps are there in your code and define where refactoring needs to be done. If these tasks are hidden, they will never be prioritized. Teams should:
- Mark PRs explicitly as “Refactoring PRs” to distinguish them from feature work.
- Maintain a technical debt backlog with well-defined tasks.
2. Make It Rewarding – Celebrate Progress
Refactoring often goes unnoticed. Recognizing and celebrating these efforts increases motivation.
- Showcase refactoring wins in sprint demos.
- Quantify improvements (e.g., 20% faster build times, 15% reduction in memory usage).
- Encourage a culture of clean coding by rewarding engineers who contribute significantly to code quality.
3. Make It Resilient – Ensure Long-Term Sustainability
Most organizations put new features above code improvement during everyday development work. While this is necessary, refactoring should never be completely deprioritized.
- Integrate technical debt tracking into sprint planning.
- Allocate dedicated refactoring time (e.g., 10-15% of sprint capacity for tech debt resolution).
- Create a roadmap for future refactoring tasks rather than assuming they can be picked up “whenever.”
Automated Tools for Technical Debt Analysis
Developers can use various automation tools to find and handle technical debt in their work. Using these tools as part of the CI/CD pipeline ensures continuous monitoring and early detection of technical debt. Some popular tools include:
- SonarQube: SonarQube helps developers find code issues like bad code design, security risks, and code repetitions.
- CodeClimate: CodeClimate helps developers perform automatic code checking while monitoring project technical debt.
- NDepend: NDepend evaluates .NET code to deliver precise assessment results.
- ESLint: ESLint finds and solves problems in JavaScript coding projects.
Metrics-Driven Refactoring
Refactoring without metrics makes it harder to track how far you’ve come in tackling technical debts. That contradicts the main agenda of your work, so it’s important that you measure your progress to identify areas needing optimization.
- Cyclomatic Complexity: Measures independent code paths; lower values improve readability and testability.
- Maintainability Index: Scores code complexity and documentation; a low score signals high maintenance effort.
- Code Churn: Tracks frequent modifications; unstable code may need better design.
Technical debt isn’t inherently bad. It allows quick development but can be problematic if neglected. Continuous refactoring helps teams to handle and minimize technical debt without breaking feature development. Regular refactoring leads to software that boasts high quality and maintainability, making it essential for long-term success.
Frequently Asked Questions
Q1: What is Technical Debt and Refactoring in Extreme Programming?
Technical debt in Extreme Programming refers to suboptimal code that slows future development, while refactoring is the process of improving code without changing functionality.
Q2: What is the Difference Between Rebuild and Refactor?
Rebuilding means rewriting an application from scratch, whereas refactoring improves existing code incrementally without changing its external behavior.
Q3: What is an Example of Technical Debt?
Example of technical debt: Hardcoded values instead of configuration files create technical debt, making future updates harder and increasing maintenance costs.